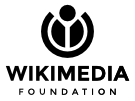
Error
Too Many Requests
If you report this error to the Wikimedia System Administrators, please include the details below.
Request served via cp3070 cp3070, Varnish XID 1024551345
Upstream caches: cp3070 int
Error: 429, Too Many Requests at Thu, 01 May 2025 00:36:31 GMTSensitive client information
IP address: 149.154.157.172